Introducing Helios for Kotlin
by Adrian Ramirez Fornell
- •
- June 12, 2019
- •
- kotlin• functional• arrow• helios• json
- |
- 3 minutes to read.
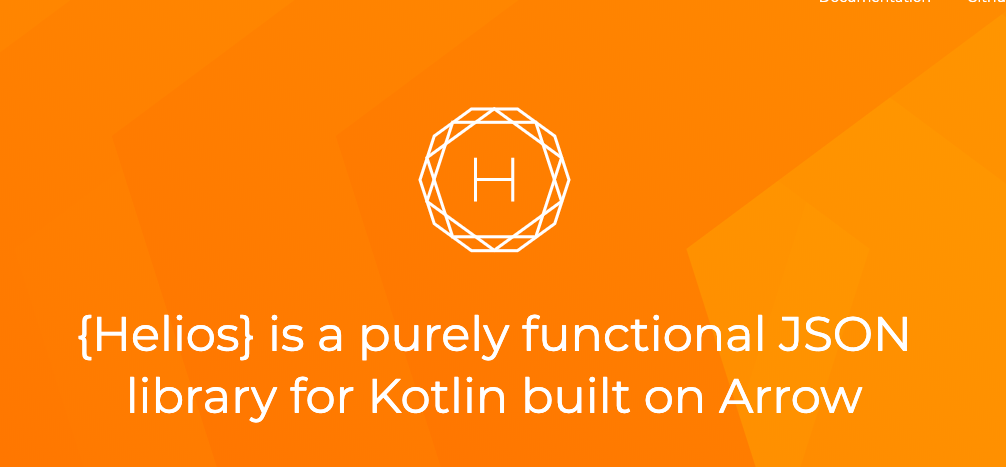
Helios is a library for JSON
handling that’s compatible with all of Kotlin
’s types including nullable types and data classes
, with few Java
types like UUID
and BigDecimal
and, also, with some Arrow
data types, Option
, Either
, and Tuple
for example.
Let’s take a brief look at how you can start using Helios:
Adding the dependency
First off, we need to add the Helios dependency to our project. We can do it by adding the following block to our build.gradle
:
repositories {
maven { url "https://jitpack.io" }
}
dependencies {
compile "com.47deg:helios-core:0.1.0"
compile "com.47deg:helios-parser:0.1.0"
compile "com.47deg:helios-optics:0.1.0"
}
Encoding and Decoding
After adding the dependency, we can start creating our little model:
@json
data class Person(val name: String, val age: Int) {
companion object
}
With the @json
annotation, we are able to automatically generate encoders and decoders for the Person
data class at compile time as follows:
val person = Person("Cristobal", 37)
val jsonFromPerson: Json = with(Person.encoder()) {
person.encode()
}
Person.decoder().decode(jsonFromPerson)
You can also write your own encoders and decoders.
The DSL
We have a simple DSL
that takes advantage of the pretty kotlin
syntax for creating your own JSON
:
import helios.core.Json
val json: Json = JsObject(
"name" to JsString("Simon"),
"age" to JsNumber(26)
)
Parsing JSON
Of course, we can parse a JSON
from an String
or a File
.
Using all of the power from the Arrow
library, we’re able to handle parsing errors:
val jsonStr =
"""{
"name": "Cristobal",
"age": 37
}"""
val jsonOrError: Either<Throwable, Json> = Json.parseFromString(jsonStr)
val json : Json = jsonOrError.getOrHandle { JsString("") }
Navigation through JSON
The optics module allows you to query and modify JSON
with compile-time safety and without any boilerplate.
import helios.optics.*
Json.path.select("name").string.modify(json, String::toUpperCase)
Note that the code generation will give you an accessor for each JSON
field.
Json.path.name.string.modify(json, String::toUpperCase)
Both will produce the same:
{
"name": "CRISTOBAL",
"age": 37
}
Resources
If you want to learn more, please take a look at Helios’ documentation, we also have an examples module that will be helpful.
If you have any questions or comments, feel free to reach out to us on the Helios slack channel or submit an issue to the Helios repository.
This is just the first release of Helios; we have plans for more awesome features like Java time
support and other integrations.
The active development of Helios is proudly sponsored by 47 Degrees, a Functional Programming consultancy with a focus on the Scala, Kotlin, and Swift Programming languages.